Home
Roadmap
Downloads 
Samples
About |
Samples
-
around1.cs
A simple demostration for around advice.
using System;
namespace AroundSample
{
public aspect AroundAspect
{
pointcut MyPointCut int MainCls.Sum(int i);
around int MyPointCut(int i)
{
Console.WriteLine("Inside Around");
Console.WriteLine("Before SUM");
int result = proceed(i);
Console.WriteLine("After SUM");
Console.WriteLine("Value in around: " + result);
return result;
}
}
public class MainCls
{
public int Sum(int i)
{
Console.WriteLine("Inside Sum");
return i+1;
}
public static void Main()
{
MainCls mainCs = new MainCls();
Console.WriteLine(mainCs.Sum(1));
}
}
}
|
Program output:
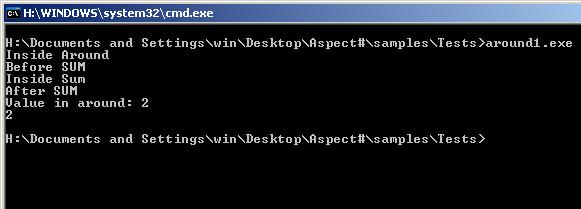
-
logging.cs
Log all methods executed in the application, the log also includes the names
and values of the parameters of the method.
using System;
using System.Reflection;
using NKalore.Model;
namespace Namespace
{
public aspect LoggingAspect
{
pointcut LogPointcut % %(...);
int level = 0;
void log(JoinPoint joinPoint, string prefix, bool showParams)
{
string pad = new string('·', level - 1);
Console.WriteLine(pad + prefix + " - " + joinPoint.MethodInfo);
if (showParams)
{
ParameterInfo[] pis = joinPoint.MethodInfo.GetParameters();
for (int i = 0; i < pis.Length; i++)
{
Console.WriteLine(pad + "\t{0} {1} = {2}",
pis[i].ParameterType, pis[i].Name, joinPoint.ParamsData[i]);
}
}
}
after LogPointcut()
{
log(thisJoinPoint, "after", false);
level--;
}
before LogPointcut()
{
level++;
log(thisJoinPoint, "before", true);
}
}
public class MainCls
{
public MainCls() { a(2); }
void a(int a) { b("paul", 14); }
void b(string name, double age) { c(true); }
void c(bool someFlag) { return; }
public static void Main()
{
new MainCls();
}
}
}
|
Program output:
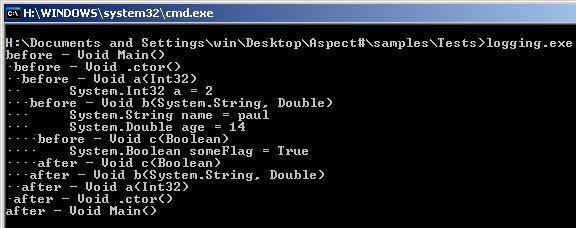
-
exception5.cs
Apply the advice throwing to catch any exception that occur in the RunCommand method, and change the result of the method.
using System;
namespace ExceptionSample
{
public aspect CatchAspect
{
pointcut MyPointCut bool %.RunCommand(int i);
throwing bool MyPointCut(Exception ex)(int i)
{
Console.WriteLine("Error: " + ex.Message);
return false;
}
}
public class MainCls
{
public bool RunCommand(int i)
{
int k = 10 / i;
Console.WriteLine("Division: " + k);
return true;
}
public static void Main()
{
MainCls mainCs = new MainCls();
bool success;
for (int i = 0; i < 3; i++)
{
Console.WriteLine("Running command: " + i);
success = mainCs.RunCommand(i);
Console.WriteLine((success? "OK": "Not OK"));
}
}
}
}
|
Program output:
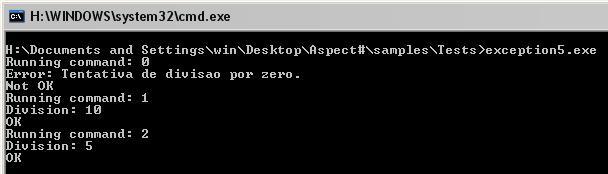
-
Constructor.cs
Apply the advice after and before in a constructor.
using System;
namespace ConsoleApplication1
{
public aspect Class2
{
pointcut MyPointCut ctor ConsoleApplication1.MainCls.MainCls();
after MyPointCut()
{
Console.WriteLine("after " + thisJoinPoint.MethodInfo);
}
before MyPointCut()
{
Console.WriteLine("before " + thisJoinPoint.MethodInfo);
}
}
public class MainCls
{
public MainCls()
{
Console.WriteLine("MainCls()");
}
public static void Main()
{
new MainCls();
}
}
}
|
Program output:
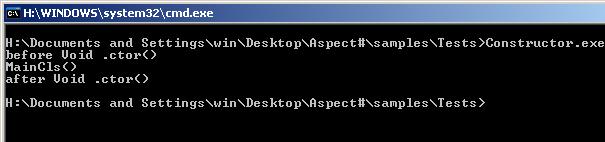
-
around3.cs
Use the around advice to cache the result of all method that return a boolean
value. So, if you can call the method twice (wth the same parameters), the
second time, the result is read from cache.
using System;
using System.Collections;
namespace AroundSample
{
public aspect AroundAspect
{
pointcut IsMethodsCache bool MainCls.Is%(...);
Hashtable cache = new Hashtable();
around bool IsMethodsCache()
{
bool result;
// makes a unique key
string key = thisJoinPoint.MethodInfo.GetHashCode().ToString();
foreach (object param in thisJoinPoint.ParamsData)
key += "." + param.GetHashCode();
if (cache[key] == null)
{
//Console.WriteLine("No cache is avaiable");
result = proceed(...);
cache[key] = result;
}
else
{
Console.WriteLine("Get result from cache");
result = (bool)cache[key];
}
return result;
}
}
public class MainCls
{
public bool IsPrime(long number)
{
for (long d = 2; d < number; d++)
{
if (number % d == 0)
{
return false;
}
}
return true;
}
public bool IsSumBiggerThanZero(int a, int b)
{
return (a + b > 0);
}
public int IsOtherThing()
{
return 0;
}
public static void Main()
{
MainCls mainCs = new MainCls();
Console.WriteLine("7 is prime = " + mainCs.IsPrime(7));
Console.WriteLine("4 is prime = " + mainCs.IsPrime(4));
Console.WriteLine("7 is prime = " + mainCs.IsPrime(7));
Console.WriteLine("4+-1 > 0 = " + mainCs.IsSumBiggerThanZero(4,-1));
Console.WriteLine("7+32 > 0 = " + mainCs.IsSumBiggerThanZero(7,32));
Console.WriteLine("4+-1 > 0 = " + mainCs.IsSumBiggerThanZero(4,-1));
}
}
}
|
Program output:
 |
|